In Codecademy, when learning the basics of JavaScript we are given a project of building Kelvin weather, where we have to convert Kelvin to Celsius, then to Fahrenheit.
In this article, I’ve explained the javascript codes with explanations.
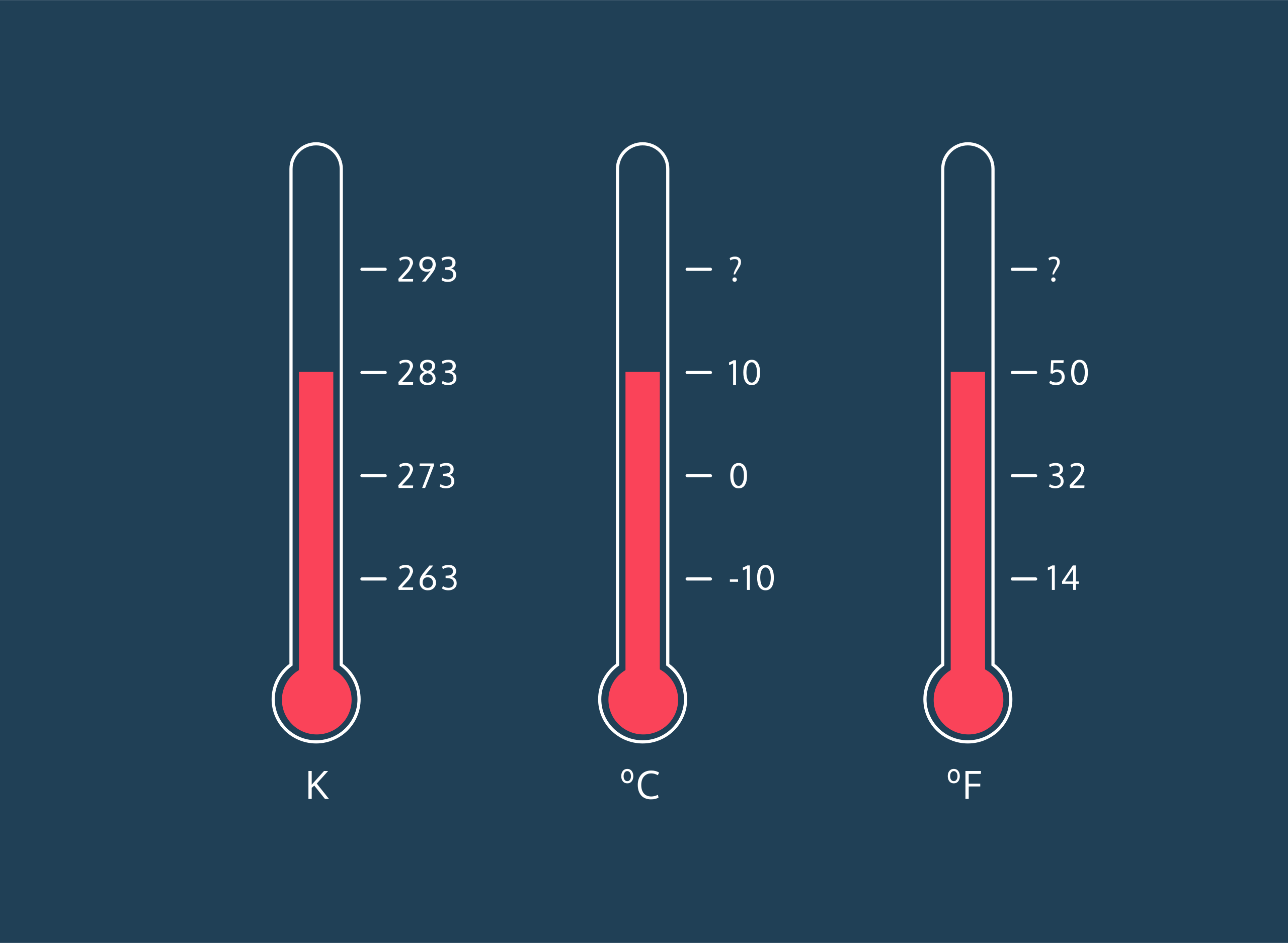
Data we’ve already with us:
- Today’s weather forecast: 293 Kelvin.
- Celsius is similar to Kelvin — the only difference is that Celsius is 273 degrees less than Kelvin.
- Fahrenheit = Celsius * (9/5) + 32
So let’s begin. Here begins the javascript code.
// We set constant value for kelvin which is 293. const kelvin = 293; // We set celsius value which is 273 less in kelvin. const celsius = kelvin - 273; // Used formula to sum fahrenheit. let fahrenheit = celsius * (9 / 5) + 32; // By using the .floor() method from the built-in Math object to round down the Fahrenheit temperature. fahrenheit = Math.floor(fahrenheit); // Outcome of above calculations. console.log(`The current temprature is ${fahrenheit} degrees fehrenheit.`);
In the last line, I have used the backtick not the apostrophe symbol, do remember that.
The outcome of the above codes should be:
The current temprature is 68 degrees fehrenheit.
This is it. That’s how we can do this Kelvin weather project in javascript.
Leave a Reply