Time is the most valuable currency in this fast running world. Boring and repetitive tasks like copying Excel data, converting PDFs, or sending status emails eat up hours that could be spent on high-impact work and can give you some rest.
What if you could automate those tasks with just a few lines of Python code? Sounds cool, right. I am saving my hours of time with this.
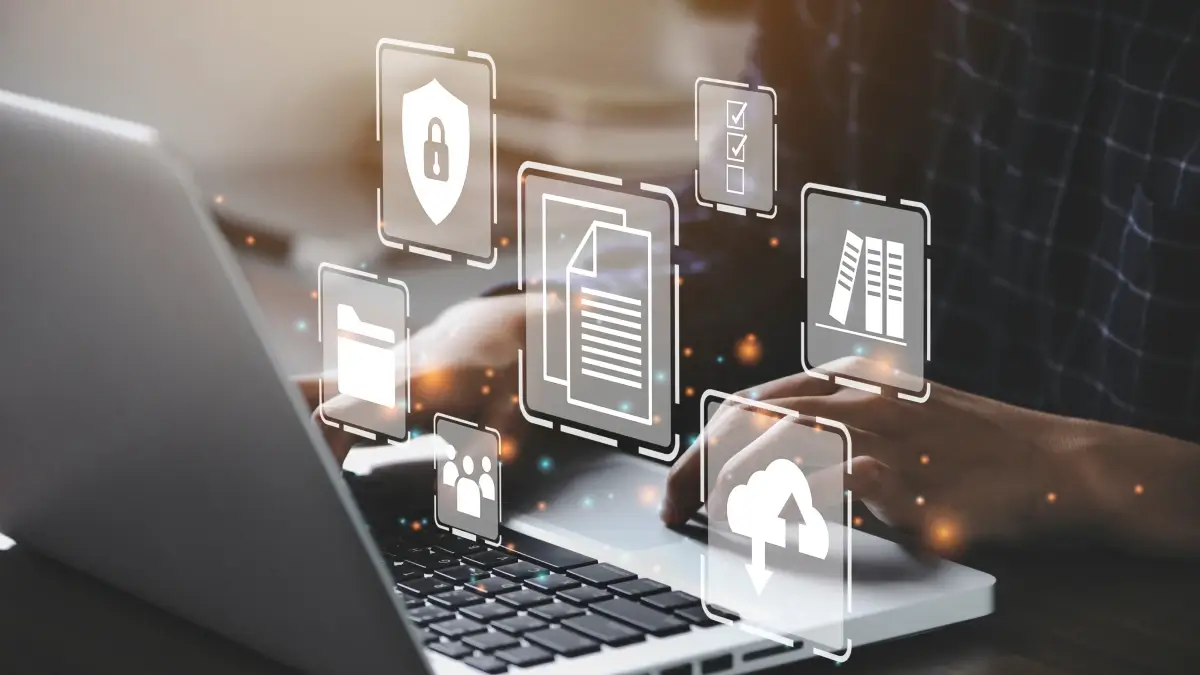
Python is more than just a language for data scientists and developers. It’s also a powerful tool for office productivity—capable of transforming the way you work by automating mundane, manual processes.
In this article, we’ll explore how to automate common office tasks using Python, including:
- Handling spreadsheet (excel) files.
- Working with PDFs.
- Sending automated emails.
- And more…
Automating Excel Tasks with openpyxl
& pandas
. 📊
Working with Excel is probably the most common office task. Whether you’re crunching numbers, tracking leads, or preparing reports—Python can do it all.
Libraries Used: openpyxl
, pandas
.
Tasks You Can Automate:
- Reading and writing data to Excel.
- Filtering and cleaning large datasets.
- Generating summary reports.
- Formatting and styling Excel sheets.
import pandas as pd
# Read an Excel file
df = pd.read_excel("sales_data.xlsx")
# Clean and analyze
df = df.dropna()
summary = df.groupby("Region")["Revenue"].sum()
# Export to a new Excel file
summary.to_excel("regional_summary.xlsx")
Use Case: Automate monthly sales reports with a script that runs in seconds instead of spending hours manually copying data.
Working with PDFs using PyPDF2
& pdfplumber
. 📄
PDFs are notoriously tricky to work with, especially when extracting text or merging documents.
Libraries Used: PyPDF2
, pdfplumber
, reportlab
.
What You Can Do:
- Merge or split PDF files
- Extract text or tables from PDFs
- Fill forms or watermark pages
import PyPDF2
# Merge two PDFs
pdf1 = open('doc1.pdf', 'rb')
pdf2 = open('doc2.pdf', 'rb')
merger = PyPDF2.PdfMerger()
merger.append(pdf1)
merger.append(pdf2)
merger.write("merged.pdf")
merger.close()
Use Case: Automatically combine client reports into a single file or extract tables from invoices for bookkeeping.
Automating Emails with smtplib
& email
. 📧
Whether it’s sending weekly updates, newsletters, or auto-replies—email automation saves serious time.
Libraries Used: smtplib
, email.message
, ssl
.
Automate Tasks Like:
- Sending bulk personalized emails
- Scheduling daily/weekly reports
- Sending attachments
import smtplib
from email.message import EmailMessage
email = EmailMessage()
email['Subject'] = "Weekly Report"
email['From'] = "your@email.com"
email['To'] = "client@email.com"
email.set_content("Hi, please find the attached report.")
with open("report.pdf", "rb") as file:
email.add_attachment(file.read(), maintype="application", subtype="pdf", filename="report.pdf")
with smtplib.SMTP_SSL('smtp.gmail.com', 465) as smtp:
smtp.login('your@email.com', 'yourpassword')
smtp.send_message(email)
Use Case: Automatically email reports to stakeholders every Friday at 9 a.m. using a scheduler like cron
or Windows Task Scheduler.
Other Handy Automation Tools in Python. 🧰
os
andshutil
– For file management tasks like renaming, moving, or backing up files.datetime
– Automate date-based operations, such as timestamping files.schedule
orAPScheduler
– Run scripts at set intervals (daily, weekly, etc.).selenium
orrequests
– Automate web tasks like filling forms or scraping data.
Bonus Tip: Combine It All. 🧠
The real magic of Python happens when you combine these tasks into full workflows. For example:
Pull data from Excel → generate a PDF report → send it via email — all in one script!
# Pseudo workflow
read_excel_data()
create_summary_pdf()
send_email_with_attachment()
Getting Started. 🚀
You don’t need to be a Python expert. Start small:
- Install Python and your favorite IDE (VS Code is great)
- Learn to use pip to install packages (
pip install pandas openpyxl
) - Explore and experiment with small scripts
Once you get the hang of it, you’ll start to see automation opportunities everywhere.
Final Thoughts.
Office automation with Python is a game-changer.
It saves time, reduces errors, and frees you up for the work that truly matters. Whether you’re in admin, HR, marketing, or finance—there’s a Python-powered solution waiting to be built for your repetitive tasks.
If you’re just getting started, pick one task and write a simple script for it. You’ll be amazed at how much time you save.
Have any office tasks you’d like to automate?
Drop a comment or reach out—let’s build something together.
Leave a Reply